Motion in 1D
Contents
Motion in 1D#
Position, velocity, and acceleration#
The position of a body as a function of time, denoted by \(x(t)\), is relative to some arbitrary chosen origin where \(x=0\).
Velocity \(v(t)\) is a measure of how rapidly and the direction which the position of the body is changing:
As \(\Delta t\to 0\):
Acceleration \(a(t)\) is a measure of how fast and in which direction the velocity is changing:
As \(\Delta t\to 0\):
Force#
A force is any interaction that changes the motion of an object. This is encapsulated by Newton’s first and second law of motion.
Newton’s first law of motion states that a body continues to move at the same velocity if not acted upon by external forces. This concept is referred to as intertia, the tendency of a body to maintain its motion when no net force acts on that body.
Newton’s second law of motion states that the acceleration of a body is proportional to the net force acting on that body, and inversely proportional to the mass of that body. In other words:
From this, the unit of force in SI units is \(kg\,m\,s^{-2}\), which is defined as Newton \((N)\).
Forces are broadly classified into contact forces, which involves physical contact between bodies, and non-contact forces, which can act at a distance. Therefore, pulling of a rope is a contact force, whereas magnetism is a non-contact force.
Gravity#
Gravitational force is a non-contact, attractive force that acts between any pair of bodies with mass. It is proportional to the mass of each body, inversely proportional to the square of the distance between the centre of those bodies, and acts in a direction that aligns with a straight line connecting the centre of the bodies. Mathematically:
where \(m_a\) is the mass of body \(A\), \(m_b\) is the mass of body \(B\), \(R_{ab}\) is the distance between the two bodies, and \(G\) is the gravitational constant equal to \(6.674 \times 10^{-11}\,m^3kg^{-1}s^{-2}\).
The force acting upon a body of unit mass m by the Earth with mass \(5.972\times10^{24}\,kg\) and radius \(6.371\times10^3\,m\) is:
where \(g=9.8\,m\,s^{-2}\), or acceleration due to gravity.
Falling objects#
Considering objects free-falling solely under gravity:
where \(x_0\) and \(v_0\) are initial position and initial velocity respectively.
First we will include libraries needed in the solutions:
import numpy as np
%matplotlib inline
import matplotlib.pyplot as plt
from sympy import Function, Symbol, diff, dsolve, pprint, integrate
from matplotlib import animation, rc
from IPython.display import HTML
Tutorial Problem 1.2#
If a rock were ejected from a volcano at an initial speed of \(200\,m/s\), what would be the maximum height that it reaches above the top of the volcano? And how long will it take to reach that height?
def x(x0, v0, t, g=9.81):
return x0 + v0*t - g*t**2/2
x0 = 0 # initial position
v0 = 200 # initial velocity
# x = 0 when rock at top of volcano
# (-9.8t**2)/2 + 200t = 0
# t = 0s or 40.8s
#choosing this time span for graph purposes
time = np.arange(0, 40.8, 0.1)
#try different time spans to check if the max value changes
position = x(x0, v0, time)
print("Max height reached = %.f m" % (max(position)))
result = np.where(position == max(position)) # find index of maximum position
print("Time needed for the body to reach that height = %.2fs" % (time[result[0][0]]))
# plot position over time
fig = plt.figure(figsize=(6,4))
plt.plot(time, position, 'k')
plt.plot(time[result[0][0]], max(position), 'ro')
plt.xlabel('time (s)')
plt.ylabel('height (m)')
plt.title('Motion of the rock over time', fontsize=14)
plt.grid(True)
plt.show()
Max height reached = 2039 m
Time needed for the body to reach that height = 20.40s
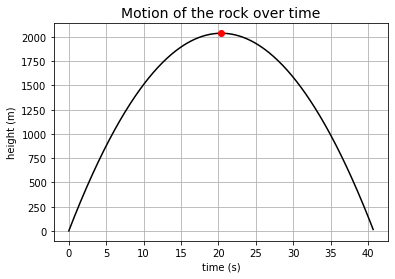
More about contact forces#
Newton’s third law of motion states that when two bodies interact, when a force is exerted from a body \(A\) to body \(B\), a force equal in magnitude and opposite in direction is exerted by body \(B\) to body \(A\).
Therefore, on the surface of contact between two bodies, there is contact force and a normal force \(N\) with the same magnitude and in opposite direction to that of the contact force.
Friction#
Friction is a contact force that always acts tangential to the contact surface between two bodies, and acts in a direction that prevents relative motion between those bodies.
The maximum friction that can be exerted is equal to \(\mu_d N\) where \(\mu_d\) is the coefficient of friction. If the force exerted in tangential direction is less than \(\mu_d N\), friction is not overcome and there won’t be relative motion.
When tangential force \(F\) is larger than \(\mu_d N\), net force on the body in the tangential direction is \(F-\mu_d N\).
Tutorial Problem 1.3#
Consider a stone of mass \(m = 24\,kg\) sitting on a flat valley floor, being hit by a gust of wind that exerts a horizontal force \(F = 5\,N\) on it for a period of time \(T = 5\,s\). Assuming the coefficient of friction between the rock and the ground is \(\mu = 0.01\), find the distance travelled by the rock before it stops sliding.
m = 24 # mass (kg)
F = 5 # force of wind (N)
T = 5 # time wind exert force on rock (s)
u = 0.01 # coefficient of friction
g = 9.81 # m/s2
time1 = np.arange(0., 5.01, 0.01) # time blown by wind
time2 = np.arange(0.01, 5.63, 0.01) # time before stopping
time = np.arange(0, 10.63, 0.01) # total amount of time
x = np.zeros(len(time))
v = np.zeros(len(time))
# Newton's second law to find acceleration
# net force = F - uN
# N = mg
# a = (F-umg)/m
# x = x0 + v0t + at**2/2
x[:501] = ((F-u*m*g)*time1**2)/(2*m) # distance over time travelled when blown by wind
# v = v0 + at
v[:501] = (F-u*m*g)*time1/m # velocity over time when blown by wind
# net force = -umg
# a = -umg/m = -ug
# x0 = last element calculated in x
# v0 = last element calculated in v
# x = x0 + v0t - at**2/2
x[501:] = x[500] + v[500]*time2 - u*g*time2**2/2 # distance over time without wind
# v = v0 + at
v[501:] = v[500] - u*g*time2 # velocity over time without wind
print("Total distance travelled = %.2fm" % (x[-1]))
# plot figure of distance and velocity of the rock over time
fig = plt.figure(figsize=(12,6))
ax1 = fig.add_subplot(121)
ax1.plot(time, x, 'r')
ax1.set_xlabel('time (s)')
ax1.set_ylabel('distance (m)')
ax1.set_ylim(0, 3)
ax1.set_title('Position of rock over time', fontsize=14)
ax1.grid(True)
ax2 = fig.add_subplot(122)
ax2.plot(time, v, 'b')
ax2.set_xlabel('time (s)')
ax2.set_ylabel('velocity (m/s)')
ax2.set_ylim(0, 0.6)
ax2.set_title('Velocity of rock over time', fontsize=14)
ax2.grid(True)
plt.show()
Total distance travelled = 2.93m
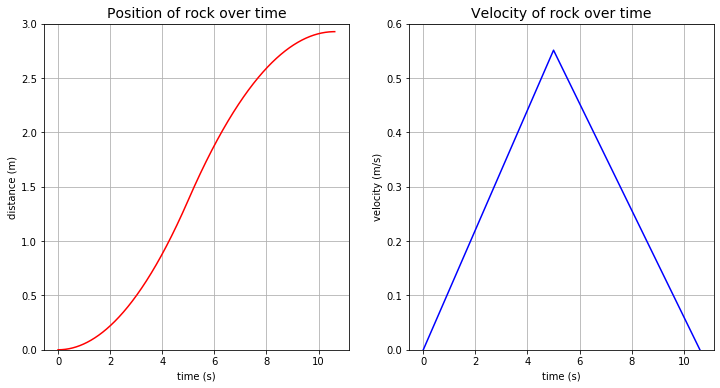
Viscous drag force#
Resistance from fluid arise from its viscosity \(\mu\), and it is called the viscous drag force.
For a spherical solid particle moving through a stationary fluid, or a fluid flowing past a stationary body, Stoke’s law states that:
where \(R\) is the radius of the particle, and \(v\) is the relative velocity between the body and the fluid. The minus sign implies it opposes the particle’s motion.
Weight of the grain \(F_w\) is given by:
where \(m_s\) is mass of the particle, \(\rho_s\) is its density, and \(V\) its volume.
Buoyancy force \(F_b\) is the weight of water displaced:
Accounting all these forces into Newton’s second law:
Rearranging this gives an ODE:
which can be solved analytically.
Tutorial Problem 1.5#
Find the terminal velocity of spherical particles with densities \(\rho_s=2.65\times10^3\,kg\,m^{-3}\), and radii \(10\,\mu m\), \(100\,\mu m\), \(1\,mm\), and \(1\,cm\).
Density of water \(\rho_w=1000\,kg\,m^{-3}\), viscosity of water \(\mu=10^{-3}\), and the velocity of a sphere from rest through a viscous fluid is given by
As \(t\to \infty\), \(v\to \frac{2(\rho_s-\rho_w)gR^2}{9\mu}\), which is the terminal velocity.
def velocity(t, R, ps=2650, pw=1000, g=9.81, mu=10e-3):
return (2*(ps-pw)*g*R**2)/(9*mu) * (1-np.exp((-9*mu*t)/(2*ps*R**2))) # analytical solution to ode
def t_v(radius, start, end, interval):
t = np.arange(start, end, interval)
v = np.zeros(len(t))
for i in range(len(t)):
v[i] = velocity(t[i], radius)
return t, v # obtain list of time and velocity for particles with different radius over a time interval
t_1cm, v_1cm = t_v(1e-2, 0, 50, 0.05)
t_1mm, v_1mm = t_v(1e-3, 0, 0.5, 0.0005)
t_10um, v_10um = t_v(10e-6, 0, 1e-4, 1e-7)
t_1um, v_1um = t_v(1e-6, 0, 5e-7, 5e-10)
print("Terminal velocity of 1um grain is %.2e m/s" % (v_1um[-1]))
print("Terminal velocity of 10um grain is %.2e m/s" % (v_10um[-1]))
print("Terminal velocity of 1mm grain is %.2f m/s" % (v_1mm[-1]))
print("Terminal velocity of 1cm grain is %.2f m/s" % (v_1cm[-1]))
# plot figures of velocity over time for grains of different radii
fig = plt.figure(figsize=(16,12))
ax1 = fig.add_subplot(221)
ax1.plot(t_1um, v_1um, 'r')
ax1.set_xlabel('time (s)')
ax1.set_ylabel('velocity (m/s)')
ax1.set_title('Velocity of sand grains with radius 1um over time')
ax1.grid(True)
ax2 = fig.add_subplot(222)
ax2.plot(t_10um, v_10um, 'y')
ax2.set_xlabel('time (s)')
ax2.set_ylabel('velocity (m/s)')
ax2.set_title('Velocity of sand grain with radius 10um over time')
ax2.grid(True)
ax3 = fig.add_subplot(223)
ax3.plot(t_1mm, v_1mm, 'g')
ax3.set_xlabel('time (s)')
ax3.set_ylabel('velocity (m/s)')
ax3.set_title('Velocity of sand grain with radius 1mm over time')
ax3.grid(True)
ax4 = fig.add_subplot(224)
ax4.plot(t_1cm, v_1cm, 'b')
ax4.set_xlabel('time (s)')
ax4.set_ylabel('velocity (m/s)')
ax4.set_title('Velocity of sand grain with radius 1cm over time')
ax4.grid(True)
plt.show()
Terminal velocity of 1um grain is 3.60e-07 m/s
Terminal velocity of 10um grain is 3.60e-05 m/s
Terminal velocity of 1mm grain is 0.36 m/s
Terminal velocity of 1cm grain is 35.96 m/s
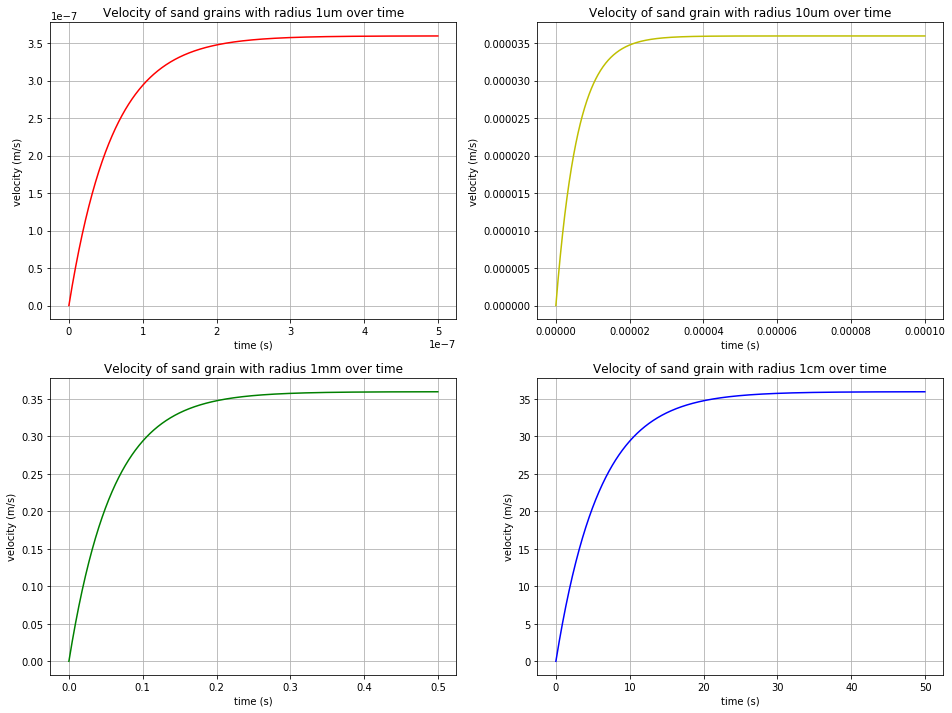
Elastic Spring Forces#
According to Hooke’s law, the force applied to the spring \(F\) is directly proportional to its extension \(x\). Mathematically:
where \(k\) is the spring constant in \(N\,m^{-1}\).
Coupled Oscillators#
Consider two masses, \(m_1\) and \(m_2\), connected by a spring with spring constant \(k\). Let the position of \(m_1\) be \(x_1\), and the position of \(m_2\) be \(x_2\).
From this, the length of the spring is \((x_2-x_1)\), and the change in length is \(\Delta(x_2-x_1)=\Delta x_2-\Delta x_1\).
Applying Hooke’s law into Newton’s second law to \(m_1\):
Applying the same laws for \(m_2\), and considering Newton’s third law:
These two equations are coupled and must be solved simultaneously.
Tutorial Problem 1.6#
The oscillatory solutions to the problem are of the form \(x_1=A_1e^{i\omega t}\), \(x_2=A_2e^{i\omega t}\). Substitute these expressions into the coupled equations, and solve the eigenvalue problem, finding the two values of \(\omega\) which are the eigenvalues, and the ratio \(\frac{A_2}{A_1}\) that corresponds to each eigenvalue.
Discuss your results.
Hint: solving the problem analytically, you should obtain the following:
When \(\omega_1=0\), \(A_1=A_2\)
When \(\omega_2=\sqrt{\frac{2k}{m}}\), \(A_1=-A_2\)
import cmath
w = np.pi
t = np.arange(0, 2*np.pi, 0.01)
X1 = np.zeros(len(t))
X2 = np.zeros(len(t))
X3 = np.zeros(len(t))
X4 = np.zeros(len(t))
for i in range(len(t)):
# w1 = 0
# A1 = A2
z1 = cmath.exp(1j*w*t[i])+1
X1[i] = z1.real
z2 = cmath.exp(1j*w*t[i])-1
X2[i] = z2.real
# w2 = (2k/m)**0.5
# A1 = -A2
# starting at different initial positions
z3 = cmath.exp(1j*w*t[i])+1
X3[i] = z3.real
z4 = -(cmath.exp(1j*w*t[i]))-1
X4[i] = z4.real
# now we make a nice animation
nframes = len(t)
# Plot background axes
fig, axes = plt.subplots(2,1, figsize=(7,5))
line1, = axes[0].plot([], [], 'ro', lw=2)
line2, = axes[0].plot([], [], 'go', lw=2)
line3, = axes[1].plot([], [], 'yo', lw=2)
line4, = axes[1].plot([], [], 'bo', lw=2)
for ax in axes:
ax.set_xlim(-2,2)
ax.set_ylim(-0.1,0.1)
axes[0].set_title('A1 = A2')
axes[1].set_title('A1 = -A2')
lines = [line1, line2, line3, line4]
plt.subplots_adjust(hspace=0.5)
# Plot background for each frame
def init():
for line in lines:
line.set_data([], [])
return lines
# Set what data to plot in each frame
def animate(i):
x1 = X1[i]
y1 = 0
lines[0].set_data(x1, y1)
x2 = X2[i]
y2 = 0
lines[1].set_data(x2, y2)
x3 = X3[i]
y3=0
lines[2].set_data(x3, y3)
x4 = X4[i]
y4 = 0
lines[3].set_data(x4, y4)
return lines
# Call the animator
anim = animation.FuncAnimation(fig, animate, init_func=init,
frames=nframes, interval=10, blit=True)
HTML(anim.to_html5_video())
References#
Course notes from Lecture 1 of the module ESE 95011 Mechanics